This post is geared toward power-users who would like to take advantage of API additions that shipped with the latest JEB update.1
TL;DR: see below for a language translation contribution in Python, and a VirusTotal hash check plugin in Java.
Contributions
With JEB 2.3.6, users can now write their own unit contribution plugins in Python (or Java, of course).
First, let’s recap: JEB extensions consist of back-end plugins, and front-end scripts. Front-end scripts are written in Python and execute in the context of a client (generally, the UI client, but it could also be a script executed by a headless, command-line JEB client). Back-end plugins form a more diverse realm: they consist of parser plugins (eg, disassemblers, decompilers, decoders, etc.), generic engines plugins, and contribution plugins. They are mostly written in Java – although that is slowly changing as we are adding program-wide support for JEB extensions in Python.
Contribution plugins can enhance the output produced by parser plugins. A concrete example: an interactive disassembly or other text output (eg, a decompiled piece of Java or C code) is made of text items; a contribution can provide additional information to a client about a given item, when the client requests it. When it comes to the main JEB UI client, that information can be requested when a user hovers its mouse over an interactive text item.
Several contributions are already built-in, such as those providing live variable and register values when debugging a program; or the Javadoc contribution that displays API documentation on Java disassembly. Users may also write their own contributions.
- Contributions extend IUnitContribution;
- They can target any type of unit;
- They can be written in Java or in Python;
- They are plugins, and as such, should be dropped into the JEB’s coreplugins/ folder (Python contributions will need a Jython package in that folder as well);
- A Python contribution must be named exactly like the contribution class name (in the above below, SampleContribution.py)
The skeleton of a Python contribution that would enhance all code units would look like:
class SampleContributionPlugin(IUnitContribution): def __init__(self): pass def getPluginInformation(self): return PluginInformation(...) def isTarget(self, unit): return isinstance(unit, ICodeUnit) def setPrimaryTarget(self, unit): self.target = unit def getPrimaryTarget(self): return self.target def getItemInformation(self, targetUnit, itemId, itemText): # provide info about an item or a bit of text def getLocationInformation(self, targetUnit, location): return None
We uploaded a sample contribution plugin that works for text documents produced by any type of parser plugin (eg, disassembly, decompiled code, etc.). The contribution uses Google to provide real-time translations of the text snippet your mouse pointer is currently on:
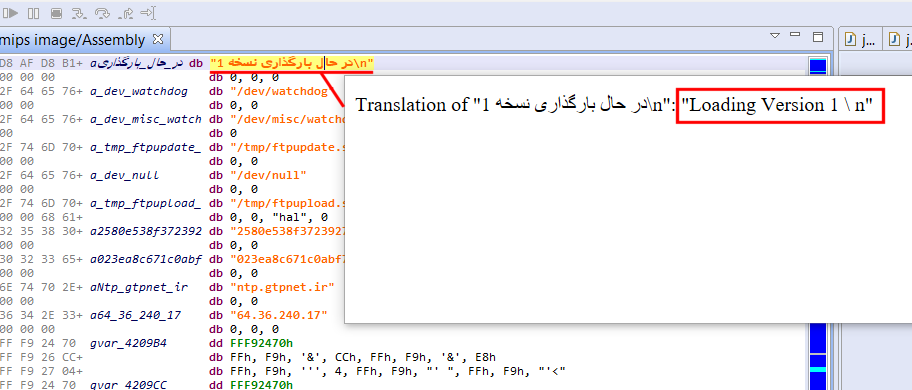
Note that you do not need a Google API key for it to work: the plugin scrapes Google search out; as such it is quite brittle and will almost certainly break in the future, but keep in mind this is a demo/sample to get you started for your own contributions.
VirusTotal Report Plugin
On a side-note, JEB 2.3.6 also ships with a VirusTotal hash checker plugin (disabled by default). This plugin automatically checks the hash of top-level units against the VirusTotal database.
We open-sourced it on GitHub (VirusTotalReportPlugin.java).
To set it up, run File, Plugins, Execute an Engines Plugin, VT Report Plugin:
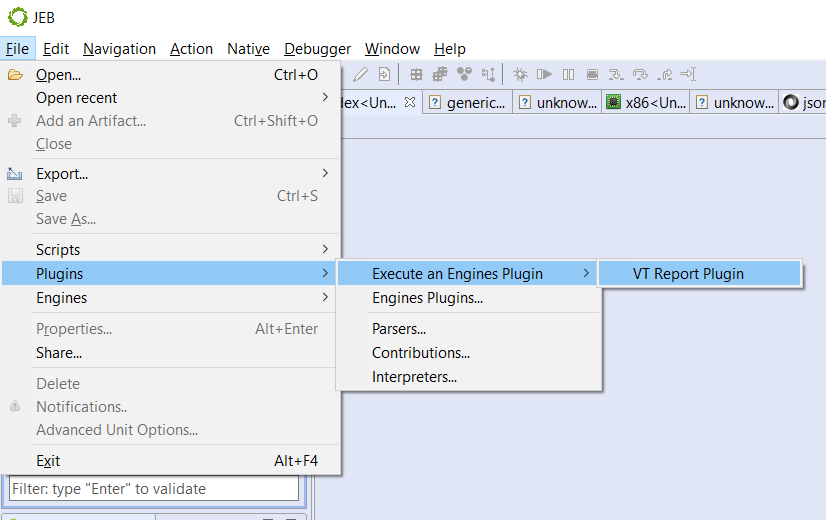
Then, enter your VirusTotal API key; you’re good to go. Newly processed files will be automatically checked against VT and a log message as well as a notification will be stored to let you know the outcome.
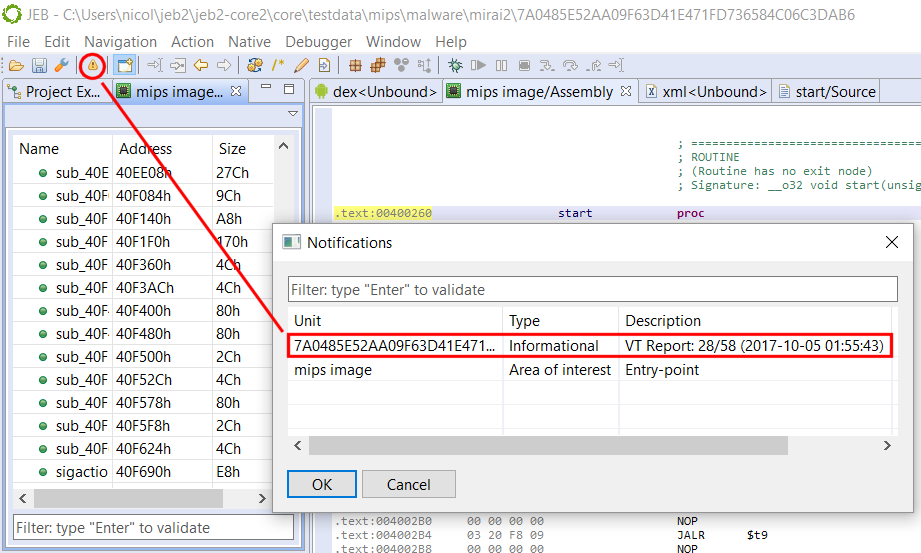
That’s it for today — until next time!
- No, we are not talking about the JEB Malware Sharing Network, that was described in the previous post! ↩